Sapiens Adaptik Platform Development Language Tutorial: A Comprehensive Guide
In the fast-paced world of insurance technology, the ability to create scalable, adaptable, and efficient applications is more important than ever. The Sapiens Adaptik platform, with its innovative capabilities, provides the tools needed to design such solutions. Central to this platform is the Sapiens Adaptik Platform Development Language Tutorial, a robust object-oriented programming language tailored for building dynamic insurance applications.
This guide will provide a detailed, step-by-step tutorial on understanding and mastering the Sapiens Adaptik Platform Development Language Tutorial. We’ll cover the platform’s key features, its development environment, syntax, best practices, and advanced techniques that will help you build industry-standard insurance applications. Whether you are just starting or looking to deepen your skills, this tutorial will provide the knowledge and insights you need to succeed.
What is the Sapiens Adaptik Platform?
Understanding the Sapiens Adaptik Platform
Before diving into the Sapiens Adaptik Platform Development Language Tutorial, it’s crucial to understand the platform itself. The Sapiens Adaptik platform is a cutting-edge technology designed specifically for the insurance industry. It enables insurers to develop, maintain, and scale digital insurance applications that meet the constantly changing demands of the market.
The platform’s main strength lies in its adaptability. It’s designed to be flexible and scalable, allowing developers to create applications that can easily evolve as business needs change. Whether it’s handling new regulatory requirements, integrating with external systems, or adapting to new customer demands, the Sapiens Adaptik platform allows insurers to stay ahead of the curve.
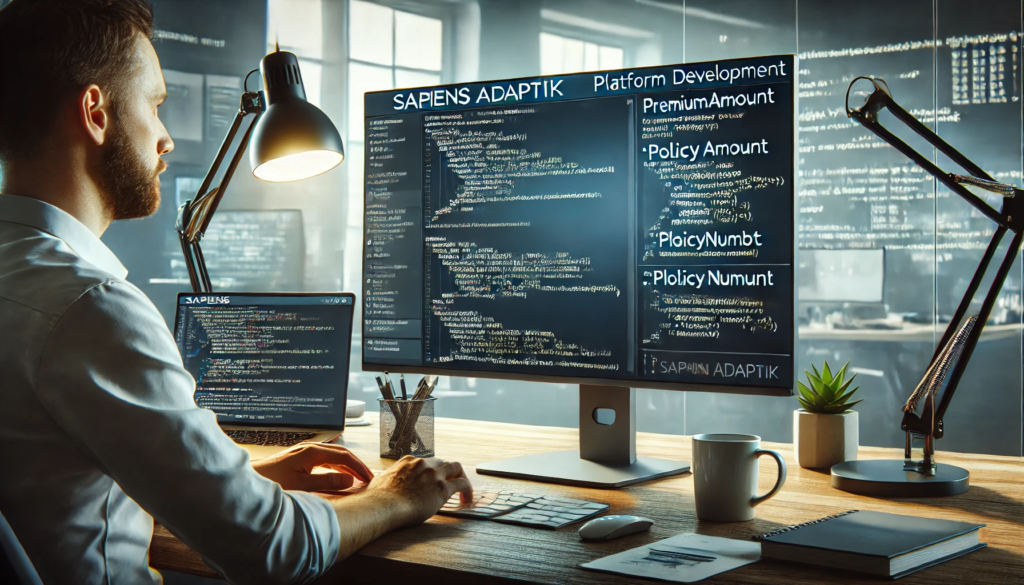
Key features of the platform include:
- Modular Architecture: This feature allows developers to design applications that can be easily updated or expanded without disrupting the overall functionality.
- Ease of Integration: The platform supports integration with a variety of third-party tools, including legacy systems and external APIs, making it an excellent choice for insurers looking to modernize their systems.
- Low-Code Environment: The Sapiens Adaptik platform supports a low-code approach to development, which helps reduce the time and effort needed to create new applications or modify existing ones.
Overall, the Sapiens Adaptik platform is designed to simplify the process of building adaptable and scalable insurance applications, making it an ideal choice for insurance companies looking to modernize their technology stack.
The Sapiens Adaptik Platform Development Language Tutorial: An Overview
Introduction to the Sapiens Adaptik Development Language
The Sapiens Adaptik Platform Development Language Tutorial is the programming language designed specifically for use with the Sapiens Adaptik platform. This language is object-oriented and is optimized to build adaptable, scalable, and efficient insurance applications. As an object-oriented programming (OOP) language, it shares similarities with other modern OOP languages, such as Java and C#, while also incorporating unique features tailored for the insurance industry.
Also Read: KVCORE: A Comprehensive Look at the Leading Real Estate CRM Solution
Why Use the Sapiens Adaptik Language?
The Sapiens Adaptik Platform Development Language Tutorial offers several advantages that make it particularly well-suited for the creation of insurance applications:
- Tailored to Insurance Needs: The language comes with built-in libraries and frameworks specifically designed for common insurance processes like underwriting, claims management, and policy administration.
- Modularity: The language allows developers to create modular, reusable code that can be easily adapted to meet changing business needs. This is particularly important in the insurance industry, where regulatory and market demands are constantly evolving.
- Cross-Platform Compatibility: Applications built using the Sapiens Adaptik language can run across different platforms, ensuring seamless integration with existing enterprise systems.
- Error Handling and Debugging: The language includes robust tools for handling errors and debugging, ensuring that applications run smoothly and are easy to maintain.
In short, the Sapiens Adaptik Platform Development Language Tutorial is optimized for building robust insurance applications that can be adapted and scaled to meet business needs. It provides a powerful and flexible development environment that speeds up the process of application development while ensuring high-quality results.
Setting Up Your Development Environment
Preparing for Development: Tools and Software Requirements
To start developing applications on the Sapiens Adaptik platform, you need to set up your development environment. The platform works with multiple IDEs (Integrated Development Environments) that facilitate efficient coding, testing, and debugging. Here’s what you need to get started:
1. Install the Sapiens Adaptik Platform
The first step in setting up your development environment is installing the Sapiens Adaptik platform. This typically involves downloading and configuring the platform on your local machine or server. Sapiens provides detailed installation instructions, which may include setting up required dependencies, database configurations, and necessary runtime environments.
Also Read: ADP Run: A Step-by-Step Payroll Solution
Once the installation is complete, you’ll have access to the platform’s development tools, including:
- Adaptik Designer: A tool for designing insurance workflows and rules.
- Adaptik Engine: The backend engine that processes business logic and integrates with other systems.
- Adaptik Studio: A full-featured IDE for writing and managing code in the Sapiens Adaptik language.
2. Choose an IDE
The Sapiens Adaptik platform works seamlessly with several IDEs, including:
- Eclipse: A widely used IDE for Java-based development, which also supports the Sapiens Adaptik platform.
- IntelliJ IDEA: A powerful IDE that offers excellent support for object-oriented programming languages like the Sapiens Adaptik language.
Both IDEs offer advanced features such as code autocompletion, syntax highlighting, and error detection, which significantly enhance productivity and reduce the likelihood of bugs.
3. Set Up Version Control
Using version control is a best practice when developing applications, and it’s no different with the Sapiens Adaptik platform. Git is the most commonly used version control system in software development, and it helps you track changes to your code, collaborate with team members, and manage different versions of your application.
Setting up a Git repository is essential for maintaining the integrity of your codebase, particularly as your project grows in complexity.
Understanding the Syntax of the Sapiens Adaptik Language
Learning the Syntax and Structure
The Sapiens Adaptik Platform Development Language Tutorial is object-oriented, which means it’s based on classes and objects. It also follows many common programming practices, but with syntax and features tailored specifically for insurance applications. Here are some key concepts:
1. Classes and Objects
In the Sapiens Adaptik Platform Development Language Tutorial, a class is a blueprint for creating objects. Each class can contain:
- Properties: These are the characteristics of an object (e.g., policyNumber, claimAmount, customerName).
- Methods: These are functions that define the actions or behaviors of an object (e.g., calculatePremium(), processClaim()).
For instance, you might create a Policy class like this:
java
CopyEdit
class Policy {
String policyNumber;
double premiumAmount;
void calculatePremium() {
// Logic to calculate premium based on risk factors
}
void updatePolicyDetails() {
// Logic to update policy details
}
}
2. Data Types
The Sapiens Adaptik language supports various data types, including primitive types (e.g., int, double, String) and complex types (e.g., arrays, lists, maps). Understanding how to use data types is crucial for storing and manipulating data in your applications.
Also Read: IASSC Universally Accepted Lean Six Sigma Bodies of Knowledge Example: A Comprehensive Guide
3. Error Handling
The Sapiens Adaptik language includes robust error-handling mechanisms to ensure your applications are reliable. You can use try-catch blocks to catch and handle exceptions that occur during execution.
Example of error handling:
java
CopyEdit
try {
// Code that might throw an exception
calculatePremium();
} catch (Exception e) {
// Handle the exception
logError(e);
}
Creating Your First Application
Building a Basic Insurance Application
Now that you have a basic understanding of the Sapiens Adaptik Platform Development Language Tutorial, it’s time to put your knowledge into practice. In this section, we’ll guide you through the process of building a simple insurance application that can calculate insurance premiums.
Step-by-Step Guide:
- Define Your Classes: Start by defining the core classes that represent different components of your application. For example, a Policy class that holds policy information and a Customer class that holds customer details.
- Define Methods: Add methods to each class to implement the business logic. For example, the calculatePremium() method in the Policy class would contain the logic for calculating premiums based on various risk factors.
- Create Relationships Between Classes: In an insurance application, classes often interact with one another. For example, a Claim class might need to reference a Policy to process a claim.
- Implement Business Logic: Use the platform’s built-in features to implement complex business logic, such as underwriting rules or claims processing.
- Test Your Application: After writing your code, it’s crucial to test your application thoroughly to ensure it works as expected. The Sapiens Adaptik platform provides testing tools to help you simulate different scenarios and identify potential issues before going live.
Best Practices for Sapiens Adaptik Development
Writing Efficient and Maintainable Code
As with any programming language, following best practices will help ensure your code is efficient, maintainable, and scalable. Here are some essential best practices when developing with the Sapiens Adaptik Platform Development Language Tutorial:
- Keep Code Modular: Break down your code into smaller, reusable modules. This will make your code easier to maintain and update.
- Leverage Inheritance and Polymorphism: These core principles of object-oriented programming help reduce code duplication and improve flexibility.
- Follow Naming Conventions: Use consistent naming conventions for classes, methods, and variables. This will make your code easier to read and understand.
- Document Your Code: Comment your code thoroughly to explain complex logic or decisions made during development.
- Test Early and Often: Write unit tests for your code and run them frequently to catch errors early in the development process.
Also Read: Canvas KISD: A Comprehensive Guide to the Digital Learning Platform for Killeen ISD
FAQs About the Sapiens Adaptik Platform Development Language Tutorial
What makes the Sapiens Adaptik Platform Development Language Tutorial different from other programming languages?
The Sapiens Adaptik Platform Development Language Tutorial is tailored for the insurance industry, offering features like built-in support for insurance workflows and integration capabilities with third-party systems. It is optimized for building scalable and adaptable insurance applications.
How do I integrate my Sapiens Adaptik application with existing systems?
The platform supports seamless integration with various legacy systems, external APIs, and third-party tools. Developers can use the platform’s integration tools to connect with different systems without disrupting business operations.
Can I use the Sapiens Adaptik platform for non-insurance applications?
While the Sapiens Adaptik platform is specifically designed for the insurance industry, its flexible architecture allows it to be adapted for other use cases. However, its full potential is realized when building insurance-specific applications.
Is the Sapiens Adaptik platform suitable for large-scale applications?
Yes, the platform is designed to handle large-scale applications, with features like scalability, modularity, and the ability to process large volumes of data efficiently.
Conclusion
The Sapiens Adaptik Platform Development Language Tutorial provides a powerful, scalable, and flexible environment for building insurance applications. Whether you’re a beginner or an experienced developer, this comprehensive tutorial has equipped you with the knowledge and tools to get started. By following best practices and understanding the core features of the platform, you’ll be able to create adaptable and efficient insurance solutions that meet the needs of the modern industry.